Java String Split Example
I don't know how many times I need to Split String in Java. Split is very common operation given various data sources e.g CSV file which contains input string in form of large String separated by comma. Splitting is necessary and Java API has great support for it. Java provides two convenience methods to split strings first within the java.lang.String class itself: split (regex) and other in java.util.StringTokenizer. Both are capable to split the string by any delimiter provided to them. Since String is final in Java every split-ed String is a new String in Java.

How to replace String in Java, convert String to Date in Java or convert String to Integer in Java. If you feel enthusiastic about String and you can also learn some bits from those post. If you like to read interview articles about String in Java you can see :
String Split Example Java
Let's see an example of splitting string in Java by using split() function:
//split string example
String assetClasses = "Gold:Stocks:Fixed Income:Commodity:Interest Rates";
String[] splits = asseltClasses.split(":");
System.out.println("splits.size: " + splits.length);
for(String asset: assetClasses){
System.out.println(asset);
}
OutPut
splits.size: 5
Gold
Stocks
Fixed Income
Commodity
Interest Rates
In above example we have provided delimiter or separator as “:” to split function which expects a regular expression and used to split the string.
Now let see another example of split using StringTokenizer
//string split example StringTokenizer
StringTokenizer stringtokenizer = new StringTokenizer(asseltClasses, ":");
while (stringtokenizer.hasMoreElements()) {
System.out.println(stringtokenizer.nextToken());
}
OutPut
Gold
Stocks
Fixed Income
Commodity
Interest Rates
How to Split Strings in Java – 2 Examples
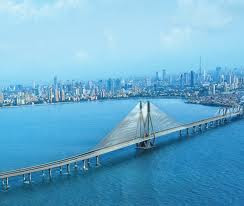
My personal favorite is String.split () because it’s defined in String class itself and its capability to handle regular expression which gives you immense power to split the string on any delimiter you ever need. Though it’s worth to remember following points about split method in Java
1) Some special characters need to be escaped while using them as delimiters or separators e.g. "." and "|".
//string split on special character “|”
String assetTrading = "Gold Trading|Stocks Trading|Fixed Income Trading|Commodity Trading|FX trading";
String[] splits = assetTrading.split("\\|"); // two \\ is required because "\" itself require escaping
for(String trading: splits){
System.out.println(trading);
}
OutPut:
Gold Trading
Stocks Trading
Fixed Income Trading
Commodity Trading
FX trading
// split string on “.”
String smartPhones = "Apple IPhone.HTC Evo3D.Nokia N9.LG Optimus.Sony Xperia.Samsung Charge”;
String[] smartPhonesSplits = smartPhones.split("\\.");
for(String smartPhone: smartPhonesSplits){
System.out.println(smartPhone);
}
OutPut:
Apple IPhone
HTC Evo3D
Nokia N9
LG Optimus
Sony Xperia
Samsung Charge
2) You can control number of split by using overloaded version split (regex, limit). If you give limit as 2 it will only creates two strings. For example in following example we could have total 4 splits but if we just want to create 2 we can use limit.
//string split example with limit
String places = "London.Switzerland.Europe.Australia";
String[] placeSplits = places.split("\\.",2);
System.out.println("placeSplits.size: " + placeSplits.length );
for(String contents: placeSplits){
System.out.println(contents);
}
Output:
placeSplits.size: 2
London
Switzerland.Europe.Australia
To conclude the topic StringTokenizer is old way of tokenizing string and with introduction of split since JDK 1.4 its usage is discouraged. No matter what kind of project you work you often need to split string in Java so better get familiar with these API.
Related Java tutorials
Tidak ada komentar:
Posting Komentar